|
| 1 | +# react-native-tm |
| 2 | +Fully customizable toast component for your react-native applications supported on IOS and Android. Also you can use it with expo or pure react-native. |
| 3 | + |
| 4 | +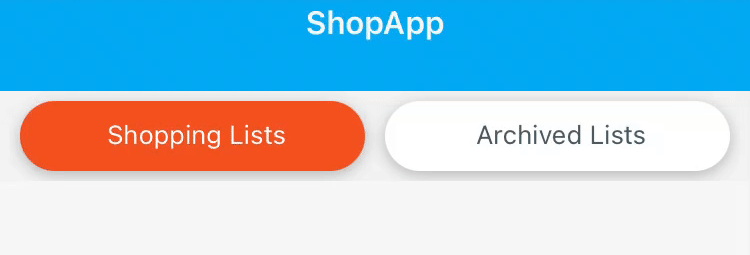 |
| 5 | + |
| 6 | +# Installation |
| 7 | +expo: `expo install react-native-tm` |
| 8 | +npm: `npm i react-native-tm` |
| 9 | +yarn: `yarn add react-native-tm` |
| 10 | + |
| 11 | +## Basic usage |
| 12 | +```JS |
| 13 | +import Toast from "react-native-tm"; |
| 14 | + |
| 15 | +export default function YourComponent() { |
| 16 | + return( |
| 17 | + <YourComponentsHere></YourComponentsHere> |
| 18 | + <Toast |
| 19 | + show={true} |
| 20 | + withClose={true} |
| 21 | + style={{ |
| 22 | + toast: { |
| 23 | + width: '100%', |
| 24 | + height: 50, |
| 25 | + backgroundColor: 'red' |
| 26 | + } |
| 27 | + }} |
| 28 | + /> |
| 29 | + ) |
| 30 | +} |
| 31 | + |
| 32 | +// more about customizing below |
| 33 | +``` |
| 34 | + |
| 35 | +## How customize your toast ? |
| 36 | +```JS |
| 37 | +import Toast from "react-native-tm"; |
| 38 | + |
| 39 | +export default function YourComponent() { |
| 40 | + |
| 41 | + return( |
| 42 | + <YourComponentsHere></YourComponentsHere> |
| 43 | + <Toast |
| 44 | + show={true} |
| 45 | + // set the animation type of toast choose the best for you in props |
| 46 | + animationType={'bounce'} |
| 47 | + // add the closing toast function on press |
| 48 | + withClose={true} |
| 49 | + // pass toast styles object to style |
| 50 | + style={{ |
| 51 | + toast: { |
| 52 | + width: '100%', |
| 53 | + height: 50, |
| 54 | + backgroundColor: 'red' |
| 55 | + } |
| 56 | + }} |
| 57 | + > |
| 58 | + // and for sure you can add childrens here |
| 59 | + // to customize your toast |
| 60 | + <View style={{height: 50, width: 50, backgroundColor: 'black', borderRadius: 30}}/> |
| 61 | + <View |
| 62 | + style={{ |
| 63 | + marginLeft: 10 |
| 64 | + }} |
| 65 | + > |
| 66 | + <Text> |
| 67 | + Title top |
| 68 | + </Text> |
| 69 | + <Text> |
| 70 | + Description on the bottom |
| 71 | + </Text> |
| 72 | + </View> |
| 73 | + </Toast> |
| 74 | + ) |
| 75 | +} |
| 76 | + |
| 77 | +``` |
| 78 | + |
| 79 | +## How customize your animation ? |
| 80 | + |
| 81 | +By default toast use the linear animation, just show and hide nothing special. But if you want to change the animation type use description below. |
| 82 | + |
| 83 | +#### For bounce animation. |
| 84 | + |
| 85 | +<table> |
| 86 | +<tr> |
| 87 | +<td> |
| 88 | + |
| 89 | +```JS |
| 90 | + <Toast |
| 91 | + ... |
| 92 | + // Add the animation type bounce |
| 93 | + animationType={'bounce'} |
| 94 | + ... |
| 95 | + /> |
| 96 | +``` |
| 97 | + |
| 98 | +</td> |
| 99 | +<td> |
| 100 | +<img src="https://user-images.githubusercontent.com/47904385/120920413-edc30900-c6be-11eb-82bf-9aa5b31dd1c8.gif" alt="drawing" width="600" height="300"/> |
| 101 | +</td> |
| 102 | +</tr> |
| 103 | +</table> |
| 104 | + |
| 105 | +#### For elastic animation. |
| 106 | + |
| 107 | +<table> |
| 108 | +<tr> |
| 109 | +<td> |
| 110 | + |
| 111 | +```JS |
| 112 | + <Toast |
| 113 | + ... |
| 114 | + // Add the animation type elastic |
| 115 | + animationType={'elastic'} |
| 116 | + ... |
| 117 | + /> |
| 118 | +``` |
| 119 | + |
| 120 | +</td> |
| 121 | +<td> |
| 122 | +<img src="https://user-images.githubusercontent.com/47904385/120920546-9f623a00-c6bf-11eb-852e-867d40f3b65f.gif" alt="drawing" width="600" height="300"/> |
| 123 | +</td> |
| 124 | +</tr> |
| 125 | +</table> |
| 126 | + |
| 127 | +## Props |
| 128 | +Below are the props you can pass to the React Component. |
| 129 | + |
| 130 | +| Prop | Type | Default | Example | Description | |
| 131 | +| ------------- | ------------- | ------------- | ------------- | ------------- | |
| 132 | +| show | boolean | | show={true} | Put the toast state | |
| 133 | +| animationType | string | | animationType={'bounce'} | If you what different animations on your toast | |
| 134 | +| toastOnPress | function | | toastOnPress={() => console.log('check')} | You can add many other functions here or just navigate to other screen | |
| 135 | +| withClose | boolean | false | withClose={true} | Added posibility to close toast on press. You can use it with toastOnPress at one time. | |
| 136 | +| children | component | | ``` <Toast><YourComponent/></Toast> ``` | You can add yout own component for example messages from users in your app or internet connection notifications. | |
| 137 | +| style | object | | {toast: {backgroundColor: 'black', height: 50}} | The styles object for styling the toast details. More about styling in Custom styling step.| |
| 138 | +| showingDuration | int | 8000 | showingDuration={3000} | How much time toast will show on the screen | |
| 139 | +| statusBarHeight | int | 180 | statusBarHeight={150} | If you have a specific status bar on your device you may want to pass this props to aware some UI bugs on the device | |
| 140 | +| onHide | function | | onHide={() => yourFunctionToDoSomething()} | Function which call when toast hiding. | |
| 141 | + |
| 142 | +## ToDos |
| 143 | + |
| 144 | +1. TypeScript support. |
| 145 | +2. More animation for customizing. |
| 146 | + |
0 commit comments