You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
> This is the coding implementations of the [DSA.js book](https://books.adrianmejia.com/dsajs-data-structures-algorithms-javascript/).
8
+
9
+
> In this repository you can find classical algorithms and data structures implemented and explained in JavaScript. it can be used as a reference manual where developers can refresh specific topics before an interview or looking for ideas to solve a problem optimally.
10
+
11
+
<!-- (Check out the Time Complexity Cheatsheet) -->
This repository covers the implementation of the classical algorithms and data structures in JavaScript.
24
+
[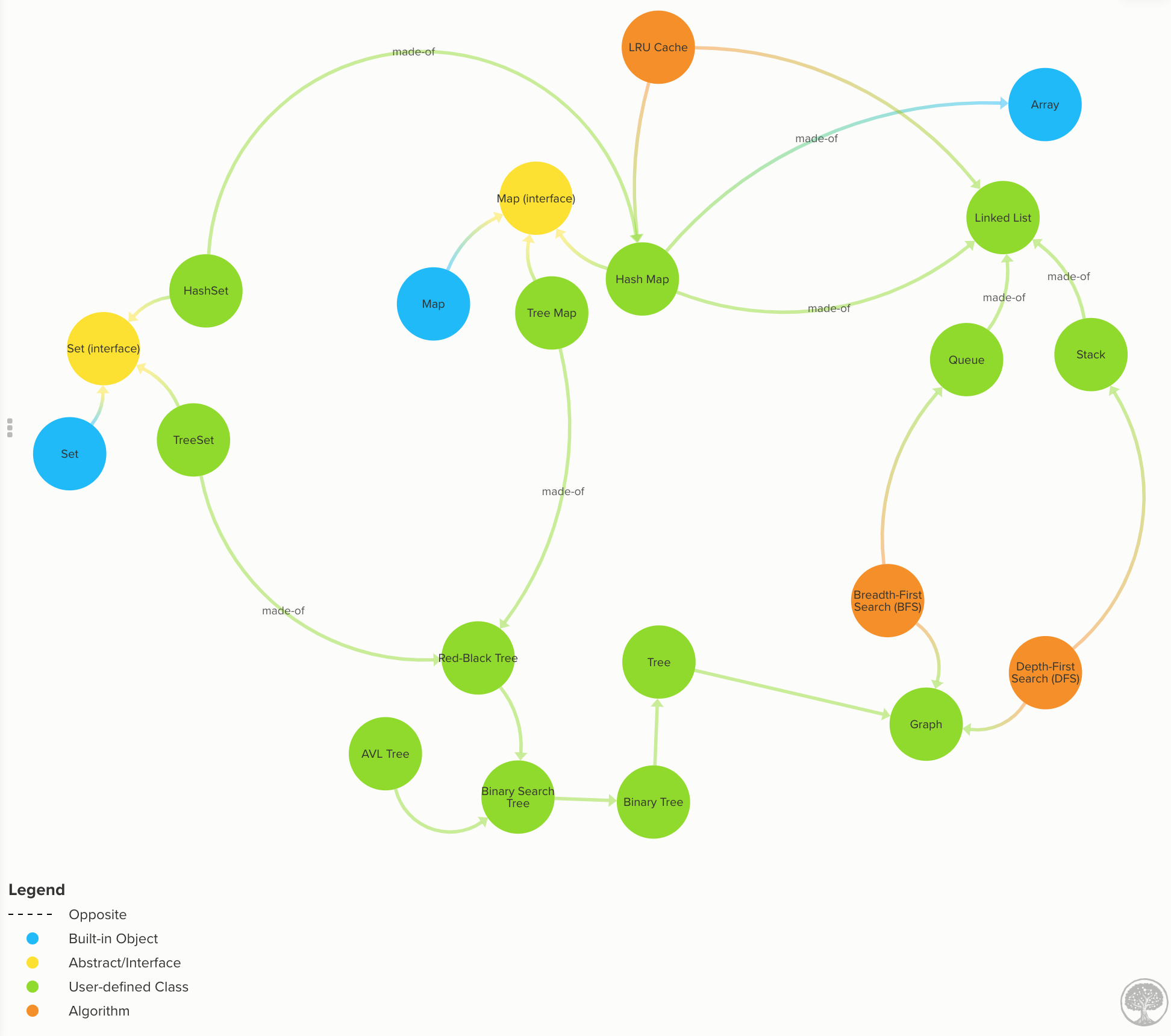](https://embed.kumu.io/85f1a4de5fb8430a10a1bf9c5118e015)
25
+
26
+
27
+
## Table of Contents (TODO)
28
+
29
+
<!-- START doctoc -->
30
+
<!-- END doctoc -->
31
+
16
32
17
-
## Usage
33
+
## Installation
18
34
19
35
You can clone the repo or install the code from NPM:
For a full list of all the exposed data structures and algorithms [see](https://github.com/amejiarosario/dsa.js/blob/master/src/index.js).
32
48
49
+
50
+
## Features
51
+
52
+
> Algorithms + Data Structures = Programs.
53
+
54
+
Algorithms are an essential toolbox for every programmer.
55
+
You usually need them when you have to sort data, search for a value, transform data, scale your code to many users and so on.
56
+
Algorithms are the step you follow to solve a problem while data structures are where you store the data.
57
+
Both combined create programs.
58
+
It's true that most programming languages and libraries provides implementations for basic data structures and algorithms.
59
+
However, to make use of data structures properly, you have to know the tradeoffs so you can choose the best tool for the job.
60
+
That's what you are going to learn here:
61
+
62
+
- 🛠 Apply strategies to tackle algorithm questions. Never to get stuck again. Ace those interviews!
63
+
- ✂️ Construct efficient algorithms. Learn how to break down problems in manageable pieces.
64
+
- 🧠 Improve your problem-solving skills and become a stronger developer by understanding fundamental computer science concepts.
65
+
- 🤓 Cover essential topics, such as big O time, data structures, and must-know algorithms. Implement 10+ data structures from scratch.
66
+
67
+
## What's Inside
68
+
69
+
### 📈 Algorithms Analysis
70
+
71
+
- Computer Science nuggets without all the mumbo-jumbo.
72
+
- Learn how to compare algorithms using Big O notation.
73
+
- 8 examples to explain with code how to calculate time complexity.
74
+
75
+
### 🥞 Linear Data Structures
76
+
77
+
- Understand the ins and outs of the most common data structures.
78
+
- When to use an Array or Linked List. Know the tradeoffs.
79
+
- Build a Stack and a Queue from scratch.
80
+
81
+
### 🌲 Non-Linear Data Structures
82
+
- Understand how hash maps and sets work.
83
+
- Know the properties of Graphs and Trees.
84
+
- Implement a binary search tree for fast lookups.
85
+
86
+
### ⚒ Algorithms Techniques
87
+
88
+
- Never get stuck solving a problem with 7 simple steps.
89
+
- Master the most popular sorting algorithms (mergesort, quicksort, insertion sort, ...)
90
+
- Learn different approaches to solve problems such as divide and conquer, dynamic programming, greedy algorithms, and backtracking.
91
+
92
+
## FAQ
93
+
94
+
<details>
95
+
<summary>How would I apply these to my day-to-day work?</summary>
96
+
<p>
97
+
As a programmer, we have to solve problems every day. If you want to solve problems well, then it's good to
98
+
know about a broad range of solutions. A lot of times, it's more efficient to learn existing resources than
99
+
stumble upon the answer yourself. The more tools and practice you have, the better. This book helps you
100
+
understand the tradeoffs among data structures and reason about algorithms performance.
101
+
</p>
102
+
</details>
103
+
104
+
<details>
105
+
<summary>Hey OP, why you created this repo/book?</summary>
106
+
<p>
107
+
There are not many books about Algorithms in JavaScript. This material fills the gap.
108
+
Also, it's good practice :)
109
+
</p>
110
+
</details>
111
+
112
+
<details>
113
+
<summary>Is there anyone I can contact if I have questions about something in particular?
114
+
</summary>
115
+
<p>
116
+
Yes, open an issue or ask questions on the slack channel.
117
+
</p>
118
+
</details>
119
+
120
+
## Support
121
+
122
+
Reach out to me at one of the following places!
123
+
124
+
- Twitter at <ahref="http://twitter.com/amejiarosario"target="_blank">`@amejiarosario`</a>
125
+
- Slack at <ahref="https://dsajs-slackin.herokuapp.com"target="_blank">`dsajs.slack.com`</a>
126
+
127
+
128
+
## Donations
129
+
130
+
The best way to support this project is buying the [book](https://books.adrianmejia.com/dsajs-data-structures-algorithms-javascript/), so I can invest more time into this project and keep improving it.
You can also buy this repo in [book format](https://books.adrianmejia.com/dsajs-data-structures-algorithms-javascript/) that goes deeper into each topic and provide additional illustrations and explanations.
0 commit comments