|
| 1 | + |
| 2 | +# Nodejs API Server |
| 3 | + |
| 4 | +Express / Nodejs Starter with JWT authentication, and **SQLite** persistance - Provided by **AppSeed** [App Generator](https://appseed.us/app-generator). |
| 5 | +Authentication Flow uses [json web tokens](https://jwt.io) via Passport library - `passport-jwt` startegy. |
| 6 | + |
| 7 | +<br /> |
| 8 | + |
| 9 | +> Features: |
| 10 | +
|
| 11 | +- Simple, intuitive codebase - can be extended with ease. |
| 12 | +- Typescript |
| 13 | +- **Stack**: NodeJS / Express / SQLite / TypeORM |
| 14 | +- Auth: Passport / `passport-jwt` strategy |
| 15 | +- [API Interface Descriptor](https://github.com/app-generator/api-server-nodejs/blob/master/media/api.postman_collection.json): POSTMAN Collection |
| 16 | + |
| 17 | +<br /> |
| 18 | + |
| 19 | +> Support: |
| 20 | +
|
| 21 | +- Github (issues tracker), Email: **support @ appseed.us** |
| 22 | +- **Discord**: [LIVE Support](https://discord.gg/fZC6hup) (registered AppSeed Users) |
| 23 | + |
| 24 | +<br /> |
| 25 | + |
| 26 | +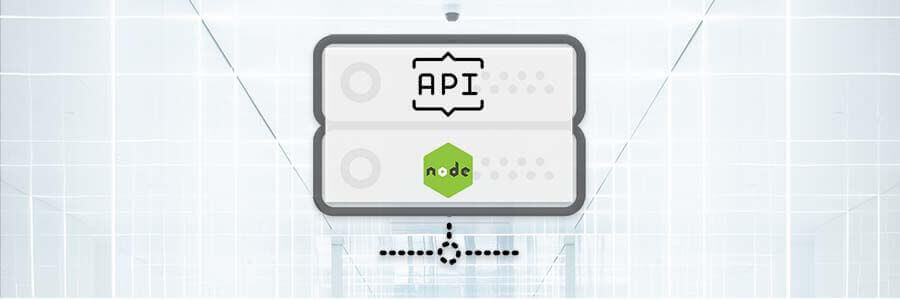 |
| 27 | + |
| 28 | +<br /> |
| 29 | + |
| 30 | +## Requirements |
| 31 | + |
| 32 | +- [Node.js](https://nodejs.org/) >= 12.x |
| 33 | +- [SQLite](https://www.sqlite.org/index.html) |
| 34 | + |
| 35 | +<br /> |
| 36 | + |
| 37 | +## How to use the code |
| 38 | + |
| 39 | +**Clone the sources** |
| 40 | + |
| 41 | +```bash |
| 42 | +$ git clone https://github.com/app-generator/api-server-nodejs.git |
| 43 | +$ cd api-server-nodejs |
| 44 | +``` |
| 45 | + |
| 46 | +**Install dependencies** via NPM or Yarn |
| 47 | + |
| 48 | +```bash |
| 49 | +$ npm i |
| 50 | +// OR |
| 51 | +$ yarn |
| 52 | +``` |
| 53 | + |
| 54 | +**Run the SQLite migration** |
| 55 | + |
| 56 | +``` |
| 57 | +$ yarn typeorm migration:run |
| 58 | +``` |
| 59 | + |
| 60 | +**Start the API server** - development mode |
| 61 | + |
| 62 | +```bash |
| 63 | +$ npm dev |
| 64 | +// OR |
| 65 | +$ yarn dev |
| 66 | +``` |
| 67 | + |
| 68 | +**Production Build** - files generated in `build` directory |
| 69 | + |
| 70 | +```bash |
| 71 | +$ npm build |
| 72 | +// OR |
| 73 | +$ yarn build |
| 74 | +``` |
| 75 | + |
| 76 | +**Start the API server** - for production (files served from `build/index.js`) |
| 77 | + |
| 78 | +```bash |
| 79 | +$ npm start |
| 80 | +// OR |
| 81 | +$ yarn start |
| 82 | +``` |
| 83 | + |
| 84 | +The API server will start using the `PORT` specified in `.env` file (default 5000) |
| 85 | + |
| 86 | +<br /> |
| 87 | + |
| 88 | +## Codebase Structure |
| 89 | + |
| 90 | +```bash |
| 91 | +< ROOT / src > |
| 92 | + | |
| 93 | + |-- config/ |
| 94 | + | |-- config.ts # Configuration |
| 95 | + | |-- passport.ts # Define Passport Strategy |
| 96 | + | |
| 97 | + |-- migration/ |
| 98 | + | |-- some_migration.ts # database migrations |
| 99 | + | |
| 100 | + |-- models/ |
| 101 | + | |-- activeSession.ts # Sessions Model (Typeorm) |
| 102 | + | |-- user.ts # User Model (Typeorm) |
| 103 | + | |
| 104 | + |-- routes/ |
| 105 | + | |-- users.ts # Define Users API Routes |
| 106 | + | |
| 107 | + | |
| 108 | + |-- index.js # API Entry Point |
| 109 | + |-- .env # Specify the ENV variables |
| 110 | + | |
| 111 | + |-- ************************************************************************ |
| 112 | +``` |
| 113 | + |
| 114 | +<br /> |
| 115 | + |
| 116 | +## SQLite Path |
| 117 | + |
| 118 | +The SQLite Path is set in `.env`, as `SQLITE_PATH` |
| 119 | + |
| 120 | +## Database migration |
| 121 | + |
| 122 | +##### generate migration: |
| 123 | + |
| 124 | +yarn typeorm migration:generate -n your_migration_name |
| 125 | + |
| 126 | +##### run migration: |
| 127 | + |
| 128 | +yarn typeorm migration:run |
| 129 | + |
| 130 | +<br /> |
| 131 | + |
| 132 | +## API |
| 133 | + |
| 134 | +For a fast set up, use this POSTMAN file: [api_sample](https://github.com/app-generator/api-server-nodejs-pro/blob/master/media/api.postman_collection.json) |
| 135 | + |
| 136 | +> **Register** - `api/users/register` |
| 137 | +
|
| 138 | +``` |
| 139 | +POST api/users/register |
| 140 | +Content-Type: application/json |
| 141 | +
|
| 142 | +{ |
| 143 | + "username":"test", |
| 144 | + "password":"pass", |
| 145 | + |
| 146 | +} |
| 147 | +``` |
| 148 | + |
| 149 | +<br /> |
| 150 | + |
| 151 | +> **Login** - `api/users/login` |
| 152 | +
|
| 153 | +``` |
| 154 | +POST /api/users/login |
| 155 | +Content-Type: application/json |
| 156 | +
|
| 157 | +{ |
| 158 | + "password":"pass", |
| 159 | + |
| 160 | +} |
| 161 | +``` |
| 162 | + |
| 163 | +<br /> |
| 164 | + |
| 165 | +> **Logout** - `api/users/logout` |
| 166 | +
|
| 167 | +``` |
| 168 | +POST api/users/logout |
| 169 | +Content-Type: application/json |
| 170 | +authorization: JWT_TOKEN (returned by Login request) |
| 171 | +
|
| 172 | +{ |
| 173 | + "token":"JWT_TOKEN" |
| 174 | +} |
| 175 | +``` |
| 176 | + |
| 177 | +<br /> |
| 178 | + |
| 179 | +## Credits |
| 180 | + |
| 181 | +This software is provided by the core AppSeed team with an inspiration from other great NodeJS starters: |
| 182 | + |
| 183 | +- Initial verison - coded by [Teo Deleanu](https://www.linkedin.com/in/teodeleanu/) |
| 184 | +- [Hackathon Starter](https://github.com/sahat/hackathon-starter) - A truly amazing boilerplate for Node.js apps |
| 185 | +- [Node Server Boilerplate](https://github.com/hagopj13/node-express-boilerplate) - just another cool starter |
| 186 | +- [React NodeJS Argon](https://github.com/creativetimofficial/argon-dashboard-react-nodejs) - released by **Creative-Tim** and [ProjectData](https://projectdata.dev/) |
| 187 | + |
| 188 | +<br /> |
| 189 | + |
| 190 | +--- |
| 191 | +Nodejs API Server - provided by AppSeed [App Generator](https://appseed.us) |
0 commit comments