|
| 1 | +// https://leetcode.com/problems/linked-list-cycle |
| 2 | +// |
| 3 | +// Given `head`, the head of a linked list, determine if the linked list has a cycle in it. |
| 4 | +// |
| 5 | +// There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the `next` pointer. Internally, `pos` is used to denote the index of the node that tail's `next` pointer is connected to. **Note that `pos` is not passed as a parameter**. |
| 6 | +// |
| 7 | +// Return `true` _if there is a cycle in the linked list_. Otherwise, return `false`. |
| 8 | +// |
| 9 | +// **Example 1:** |
| 10 | +// |
| 11 | +// 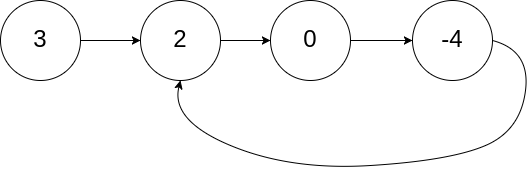 |
| 12 | +// ``` |
| 13 | +// **Input:** head = [3,2,0,-4], pos = 1 |
| 14 | +// **Output:** true |
| 15 | +// **Explanation:** There is a cycle in the linked list, where the tail connects to the 1st node (0-indexed). |
| 16 | +// ``` |
| 17 | +// |
| 18 | +// **Example 2:** |
| 19 | +// |
| 20 | +// 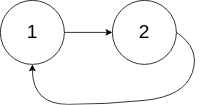 |
| 21 | +// ``` |
| 22 | +// **Input:** head = [1,2], pos = 0 |
| 23 | +// **Output:** true |
| 24 | +// **Explanation:** There is a cycle in the linked list, where the tail connects to the 0th node. |
| 25 | +// ``` |
| 26 | +// |
| 27 | +// **Example 3:** |
| 28 | +// |
| 29 | +//  |
| 30 | +// ``` |
| 31 | +// **Input:** head = [1], pos = -1 |
| 32 | +// **Output:** false |
| 33 | +// **Explanation:** There is no cycle in the linked list. |
| 34 | +// ``` |
| 35 | +// |
| 36 | +// **Constraints:** |
| 37 | +// |
| 38 | +// * The number of the nodes in the list is in the range `[0, 10<sup>4</sup>]`. |
| 39 | +// * `-10<sup>5</sup> <= Node.val <= 10<sup>5</sup>` |
| 40 | +// * `pos` is `-1` or a **valid index** in the linked-list. |
| 41 | +// |
| 42 | +// **Follow up:** Can you solve it using `O(1)` (i.e. constant) memory? |
| 43 | + |
| 44 | +export const hasCycle = function (head) { |
| 45 | + let slow = head |
| 46 | + let fast = head |
| 47 | + while (fast && fast.next) { |
| 48 | + slow = slow.next |
| 49 | + fast = fast.next.next |
| 50 | + if (slow === fast) return true |
| 51 | + } |
| 52 | + return false |
| 53 | +} |
0 commit comments