diff --git a/src/main/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/Solution.java b/src/main/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/Solution.java
new file mode 100644
index 000000000..c6f46c4e4
--- /dev/null
+++ b/src/main/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/Solution.java
@@ -0,0 +1,22 @@
+package g3401_3500.s3461_check_if_digits_are_equal_in_string_after_operations_i;
+
+// #Easy #String #Math #Simulation #Number_Theory #Combinatorics
+// #2025_02_25_Time_2_ms_(96.71%)_Space_42.26_MB_(97.03%)
+
+public class Solution {
+ public boolean hasSameDigits(String s) {
+ char[] ch = s.toCharArray();
+ int k = ch.length - 1;
+ while (k != 1) {
+ for (int i = 0; i < k; i++) {
+ int a = ch[i] - 48;
+ int b = ch[i + 1] - 48;
+ int d = (a + b) % 10;
+ char c = (char) (d + '0');
+ ch[i] = c;
+ }
+ k--;
+ }
+ return ch[0] == ch[1];
+ }
+}
diff --git a/src/main/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/readme.md b/src/main/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/readme.md
new file mode 100644
index 000000000..b8b26aabc
--- /dev/null
+++ b/src/main/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/readme.md
@@ -0,0 +1,49 @@
+3461\. Check If Digits Are Equal in String After Operations I
+
+Easy
+
+You are given a string `s` consisting of digits. Perform the following operation repeatedly until the string has **exactly** two digits:
+
+* For each pair of consecutive digits in `s`, starting from the first digit, calculate a new digit as the sum of the two digits **modulo** 10.
+* Replace `s` with the sequence of newly calculated digits, _maintaining the order_ in which they are computed.
+
+Return `true` if the final two digits in `s` are the **same**; otherwise, return `false`.
+
+**Example 1:**
+
+**Input:** s = "3902"
+
+**Output:** true
+
+**Explanation:**
+
+* Initially, `s = "3902"`
+* First operation:
+ * `(s[0] + s[1]) % 10 = (3 + 9) % 10 = 2`
+ * `(s[1] + s[2]) % 10 = (9 + 0) % 10 = 9`
+ * `(s[2] + s[3]) % 10 = (0 + 2) % 10 = 2`
+ * `s` becomes `"292"`
+* Second operation:
+ * `(s[0] + s[1]) % 10 = (2 + 9) % 10 = 1`
+ * `(s[1] + s[2]) % 10 = (9 + 2) % 10 = 1`
+ * `s` becomes `"11"`
+* Since the digits in `"11"` are the same, the output is `true`.
+
+**Example 2:**
+
+**Input:** s = "34789"
+
+**Output:** false
+
+**Explanation:**
+
+* Initially, `s = "34789"`.
+* After the first operation, `s = "7157"`.
+* After the second operation, `s = "862"`.
+* After the third operation, `s = "48"`.
+* Since `'4' != '8'`, the output is `false`.
+
+**Constraints:**
+
+* `3 <= s.length <= 100`
+* `s` consists of only digits.
\ No newline at end of file
diff --git a/src/main/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/Solution.java b/src/main/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/Solution.java
new file mode 100644
index 000000000..84b16cf91
--- /dev/null
+++ b/src/main/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/Solution.java
@@ -0,0 +1,31 @@
+package g3401_3500.s3462_maximum_sum_with_at_most_k_elements;
+
+// #Medium #Array #Sorting #Greedy #Matrix #Heap_(Priority_Queue)
+// #2025_02_25_Time_62_ms_(99.82%)_Space_78.09_MB_(20.19%)
+
+import java.util.Arrays;
+
+public class Solution {
+ public long maxSum(int[][] grid, int[] limits, int k) {
+ int l = 0;
+ for (int i = 0; i < limits.length; i++) {
+ l += limits[i];
+ }
+ int[] dp = new int[l];
+ int a = 0;
+ for (int i = 0; i < grid.length; i++) {
+ int lim = limits[i];
+ Arrays.sort(grid[i]);
+ for (int j = grid[i].length - lim; j < grid[i].length; j++) {
+ dp[a] = grid[i][j];
+ a++;
+ }
+ }
+ Arrays.sort(dp);
+ long sum = 0L;
+ for (int i = l - 1; i >= l - k; i--) {
+ sum += dp[i];
+ }
+ return sum;
+ }
+}
diff --git a/src/main/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/readme.md b/src/main/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/readme.md
new file mode 100644
index 000000000..4b9177359
--- /dev/null
+++ b/src/main/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/readme.md
@@ -0,0 +1,42 @@
+3462\. Maximum Sum With at Most K Elements
+
+Medium
+
+You are given a 2D integer matrix `grid` of size `n x m`, an integer array `limits` of length `n`, and an integer `k`. The task is to find the **maximum sum** of **at most** `k` elements from the matrix `grid` such that:
+
+* The number of elements taken from the ith
row of `grid` does not exceed `limits[i]`.
+
+
+Return the **maximum sum**.
+
+**Example 1:**
+
+**Input:** grid = [[1,2],[3,4]], limits = [1,2], k = 2
+
+**Output:** 7
+
+**Explanation:**
+
+* From the second row, we can take at most 2 elements. The elements taken are 4 and 3.
+* The maximum possible sum of at most 2 selected elements is `4 + 3 = 7`.
+
+**Example 2:**
+
+**Input:** grid = [[5,3,7],[8,2,6]], limits = [2,2], k = 3
+
+**Output:** 21
+
+**Explanation:**
+
+* From the first row, we can take at most 2 elements. The element taken is 7.
+* From the second row, we can take at most 2 elements. The elements taken are 8 and 6.
+* The maximum possible sum of at most 3 selected elements is `7 + 8 + 6 = 21`.
+
+**Constraints:**
+
+* `n == grid.length == limits.length`
+* `m == grid[i].length`
+* `1 <= n, m <= 500`
+* 0 <= grid[i][j] <= 105
+* `0 <= limits[i] <= m`
+* `0 <= k <= min(n * m, sum(limits))`
\ No newline at end of file
diff --git a/src/main/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/Solution.java b/src/main/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/Solution.java
new file mode 100644
index 000000000..ef3671d56
--- /dev/null
+++ b/src/main/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/Solution.java
@@ -0,0 +1,74 @@
+package g3401_3500.s3463_check_if_digits_are_equal_in_string_after_operations_ii;
+
+// #Hard #String #Math #Number_Theory #Combinatorics
+// #2025_02_25_Time_43_ms_(99.64%)_Space_49.40_MB_(10.02%)
+
+public class Solution {
+ private int powMod10(int a, int n) {
+ int x = 1;
+ while (n >= 1) {
+ if (n % 2 == 1) {
+ x = (x * a) % 10;
+ }
+ a = (a * a) % 10;
+ n /= 2;
+ }
+ return x;
+ }
+
+ private int[] f(int n) {
+ int[] ns = new int[n + 1];
+ int[] n2 = new int[n + 1];
+ int[] n5 = new int[n + 1];
+ ns[0] = 1;
+ for (int i = 1; i <= n; ++i) {
+ int m = i;
+ n2[i] = n2[i - 1];
+ n5[i] = n5[i - 1];
+ while (m % 2 == 0) {
+ m /= 2;
+ n2[i]++;
+ }
+ while (m % 5 == 0) {
+ m /= 5;
+ n5[i]++;
+ }
+ ns[i] = (ns[i - 1] * m) % 10;
+ }
+ int[] inv = new int[10];
+ for (int i = 1; i < 10; ++i) {
+ for (int j = 0; j < 10; ++j) {
+ if (i * j % 10 == 1) {
+ inv[i] = j;
+ }
+ }
+ }
+ int[] xs = new int[n + 1];
+ for (int k = 0; k <= n; ++k) {
+ int a = 0;
+ int s2 = n2[n] - n2[n - k] - n2[k];
+ int s5 = n5[n] - n5[n - k] - n5[k];
+ if (s2 == 0 || s5 == 0) {
+ a = (ns[n] * inv[ns[n - k]] * inv[ns[k]] * powMod10(2, s2) * powMod10(5, s5)) % 10;
+ }
+ xs[k] = a;
+ }
+ return xs;
+ }
+
+ public boolean hasSameDigits(String s) {
+ int n = s.length();
+ int[] xs = f(n - 2);
+ int[] arr = new int[n];
+ for (int i = 0; i < n; i++) {
+ arr[i] = s.charAt(i) - '0';
+ }
+ int num1 = 0;
+ int num2 = 0;
+ for (int i = 0; i < n - 1; i++) {
+ num1 = (num1 + xs[i] * arr[i]) % 10;
+ num2 = (num2 + xs[i] * arr[i + 1]) % 10;
+ }
+ return num1 == num2;
+ }
+}
diff --git a/src/main/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/readme.md b/src/main/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/readme.md
new file mode 100644
index 000000000..fe4e2de15
--- /dev/null
+++ b/src/main/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/readme.md
@@ -0,0 +1,49 @@
+3463\. Check If Digits Are Equal in String After Operations II
+
+Hard
+
+You are given a string `s` consisting of digits. Perform the following operation repeatedly until the string has **exactly** two digits:
+
+* For each pair of consecutive digits in `s`, starting from the first digit, calculate a new digit as the sum of the two digits **modulo** 10.
+* Replace `s` with the sequence of newly calculated digits, _maintaining the order_ in which they are computed.
+
+Return `true` if the final two digits in `s` are the **same**; otherwise, return `false`.
+
+**Example 1:**
+
+**Input:** s = "3902"
+
+**Output:** true
+
+**Explanation:**
+
+* Initially, `s = "3902"`
+* First operation:
+ * `(s[0] + s[1]) % 10 = (3 + 9) % 10 = 2`
+ * `(s[1] + s[2]) % 10 = (9 + 0) % 10 = 9`
+ * `(s[2] + s[3]) % 10 = (0 + 2) % 10 = 2`
+ * `s` becomes `"292"`
+* Second operation:
+ * `(s[0] + s[1]) % 10 = (2 + 9) % 10 = 1`
+ * `(s[1] + s[2]) % 10 = (9 + 2) % 10 = 1`
+ * `s` becomes `"11"`
+* Since the digits in `"11"` are the same, the output is `true`.
+
+**Example 2:**
+
+**Input:** s = "34789"
+
+**Output:** false
+
+**Explanation:**
+
+* Initially, `s = "34789"`.
+* After the first operation, `s = "7157"`.
+* After the second operation, `s = "862"`.
+* After the third operation, `s = "48"`.
+* Since `'4' != '8'`, the output is `false`.
+
+**Constraints:**
+
+* 3 <= s.length <= 105
+* `s` consists of only digits.
\ No newline at end of file
diff --git a/src/main/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/Solution.java b/src/main/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/Solution.java
new file mode 100644
index 000000000..3e6ebfe34
--- /dev/null
+++ b/src/main/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/Solution.java
@@ -0,0 +1,79 @@
+package g3401_3500.s3464_maximize_the_distance_between_points_on_a_square;
+
+// #Hard #Array #Greedy #Binary_Search #2025_02_25_Time_18_ms_(98.51%)_Space_49.78_MB_(46.27%)
+
+import java.util.Arrays;
+
+public class Solution {
+ public int maxDistance(int side, int[][] pts, int k) {
+ int n = pts.length;
+ long[] p = new long[n];
+ for (int i = 0; i < n; i++) {
+ int x = pts[i][0];
+ int y = pts[i][1];
+ long c;
+ if (y == 0) {
+ c = x;
+ } else if (x == side) {
+ c = side + (long) y;
+ } else if (y == side) {
+ c = 2L * side + (side - x);
+ } else {
+ c = 3L * side + (side - y);
+ }
+ p[i] = c;
+ }
+ Arrays.sort(p);
+ long c = 4L * side;
+ int tot = 2 * n;
+ long[] dArr = new long[tot];
+ for (int i = 0; i < n; i++) {
+ dArr[i] = p[i];
+ dArr[i + n] = p[i] + c;
+ }
+ int lo = 0;
+ int hi = 2 * side;
+ int ans = 0;
+ while (lo <= hi) {
+ int mid = (lo + hi) >>> 1;
+ if (check(mid, dArr, n, k, c)) {
+ ans = mid;
+ lo = mid + 1;
+ } else {
+ hi = mid - 1;
+ }
+ }
+ return ans;
+ }
+
+ private boolean check(int d, long[] dArr, int n, int k, long c) {
+ int len = dArr.length;
+ int[] nxt = new int[len];
+ int j = 0;
+ for (int i = 0; i < len; i++) {
+ if (j < i + 1) {
+ j = i + 1;
+ }
+ while (j < len && dArr[j] < dArr[i] + d) {
+ j++;
+ }
+ nxt[i] = (j < len) ? j : -1;
+ }
+ for (int i = 0; i < n; i++) {
+ int cnt = 1;
+ int cur = i;
+ while (cnt < k) {
+ int nx = nxt[cur];
+ if (nx == -1 || nx >= i + n) {
+ break;
+ }
+ cur = nx;
+ cnt++;
+ }
+ if (cnt == k && (dArr[i] + c - dArr[cur]) >= d) {
+ return true;
+ }
+ }
+ return false;
+ }
+}
diff --git a/src/main/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/readme.md b/src/main/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/readme.md
new file mode 100644
index 000000000..2fc14a006
--- /dev/null
+++ b/src/main/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/readme.md
@@ -0,0 +1,59 @@
+3464\. Maximize the Distance Between Points on a Square
+
+Hard
+
+You are given an integer `side`, representing the edge length of a square with corners at `(0, 0)`, `(0, side)`, `(side, 0)`, and `(side, side)` on a Cartesian plane.
+
+You are also given a **positive** integer `k` and a 2D integer array `points`, where points[i] = [xi, yi]
represents the coordinate of a point lying on the **boundary** of the square.
+
+You need to select `k` elements among `points` such that the **minimum** Manhattan distance between any two points is **maximized**.
+
+Return the **maximum** possible **minimum** Manhattan distance between the selected `k` points.
+
+The Manhattan Distance between two cells (xi, yi)
and (xj, yj)
is |xi - xj| + |yi - yj|
.
+
+**Example 1:**
+
+**Input:** side = 2, points = [[0,2],[2,0],[2,2],[0,0]], k = 4
+
+**Output:** 2
+
+**Explanation:**
+
+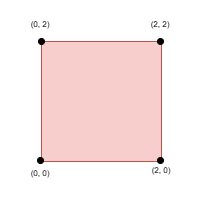
+
+Select all four points.
+
+**Example 2:**
+
+**Input:** side = 2, points = [[0,0],[1,2],[2,0],[2,2],[2,1]], k = 4
+
+**Output:** 1
+
+**Explanation:**
+
+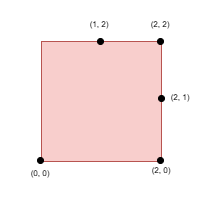
+
+Select the points `(0, 0)`, `(2, 0)`, `(2, 2)`, and `(2, 1)`.
+
+**Example 3:**
+
+**Input:** side = 2, points = [[0,0],[0,1],[0,2],[1,2],[2,0],[2,2],[2,1]], k = 5
+
+**Output:** 1
+
+**Explanation:**
+
+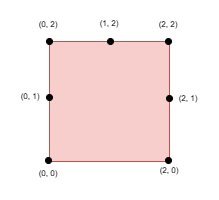
+
+Select the points `(0, 0)`, `(0, 1)`, `(0, 2)`, `(1, 2)`, and `(2, 2)`.
+
+**Constraints:**
+
+* 1 <= side <= 109
+* 4 <= points.length <= min(4 * side, 15 * 103)
+* `points[i] == [xi, yi]`
+* The input is generated such that:
+ * `points[i]` lies on the boundary of the square.
+ * All `points[i]` are **unique**.
+* `4 <= k <= min(25, points.length)`
\ No newline at end of file
diff --git a/src/test/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/SolutionTest.java b/src/test/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/SolutionTest.java
new file mode 100644
index 000000000..3156aa652
--- /dev/null
+++ b/src/test/java/g3401_3500/s3461_check_if_digits_are_equal_in_string_after_operations_i/SolutionTest.java
@@ -0,0 +1,18 @@
+package g3401_3500.s3461_check_if_digits_are_equal_in_string_after_operations_i;
+
+import static org.hamcrest.CoreMatchers.equalTo;
+import static org.hamcrest.MatcherAssert.assertThat;
+
+import org.junit.jupiter.api.Test;
+
+class SolutionTest {
+ @Test
+ void hasSameDigits() {
+ assertThat(new Solution().hasSameDigits("3902"), equalTo(true));
+ }
+
+ @Test
+ void hasSameDigits2() {
+ assertThat(new Solution().hasSameDigits("34789"), equalTo(false));
+ }
+}
diff --git a/src/test/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/SolutionTest.java b/src/test/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/SolutionTest.java
new file mode 100644
index 000000000..279e111dd
--- /dev/null
+++ b/src/test/java/g3401_3500/s3462_maximum_sum_with_at_most_k_elements/SolutionTest.java
@@ -0,0 +1,27 @@
+package g3401_3500.s3462_maximum_sum_with_at_most_k_elements;
+
+import static org.hamcrest.CoreMatchers.equalTo;
+import static org.hamcrest.MatcherAssert.assertThat;
+
+import org.junit.jupiter.api.Test;
+
+class SolutionTest {
+ @Test
+ void maxSum() {
+ assertThat(
+ new Solution().maxSum(new int[][] {{1, 2}, {3, 4}}, new int[] {1, 2}, 2),
+ equalTo(7L));
+ }
+
+ @Test
+ void maxSum2() {
+ assertThat(
+ new Solution().maxSum(new int[][] {{5, 3, 7}, {8, 2, 6}}, new int[] {2, 2}, 3),
+ equalTo(21L));
+ }
+
+ @Test
+ void maxSum3() {
+ assertThat(new Solution().maxSum(new int[][] {}, new int[] {2, 2}, 3), equalTo(0L));
+ }
+}
diff --git a/src/test/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/SolutionTest.java b/src/test/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/SolutionTest.java
new file mode 100644
index 000000000..af841e49f
--- /dev/null
+++ b/src/test/java/g3401_3500/s3463_check_if_digits_are_equal_in_string_after_operations_ii/SolutionTest.java
@@ -0,0 +1,23 @@
+package g3401_3500.s3463_check_if_digits_are_equal_in_string_after_operations_ii;
+
+import static org.hamcrest.CoreMatchers.equalTo;
+import static org.hamcrest.MatcherAssert.assertThat;
+
+import org.junit.jupiter.api.Test;
+
+class SolutionTest {
+ @Test
+ void hasSameDigits() {
+ assertThat(new Solution().hasSameDigits("3902"), equalTo(true));
+ }
+
+ @Test
+ void hasSameDigits2() {
+ assertThat(new Solution().hasSameDigits("34789"), equalTo(false));
+ }
+
+ @Test
+ void hasSameDigits3() {
+ assertThat(new Solution().hasSameDigits("3506677"), equalTo(false));
+ }
+}
diff --git a/src/test/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/SolutionTest.java b/src/test/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/SolutionTest.java
new file mode 100644
index 000000000..a7b779b2d
--- /dev/null
+++ b/src/test/java/g3401_3500/s3464_maximize_the_distance_between_points_on_a_square/SolutionTest.java
@@ -0,0 +1,36 @@
+package g3401_3500.s3464_maximize_the_distance_between_points_on_a_square;
+
+import static org.hamcrest.CoreMatchers.equalTo;
+import static org.hamcrest.MatcherAssert.assertThat;
+
+import org.junit.jupiter.api.Test;
+
+class SolutionTest {
+ @Test
+ void maxDistance() {
+ assertThat(
+ new Solution().maxDistance(2, new int[][] {{0, 2}, {2, 0}, {2, 2}, {0, 0}}, 4),
+ equalTo(2));
+ }
+
+ @Test
+ void maxDistance2() {
+ assertThat(
+ new Solution()
+ .maxDistance(2, new int[][] {{0, 0}, {1, 2}, {2, 0}, {2, 2}, {2, 1}}, 4),
+ equalTo(1));
+ }
+
+ @Test
+ void maxDistance3() {
+ assertThat(
+ new Solution()
+ .maxDistance(
+ 2,
+ new int[][] {
+ {0, 0}, {0, 1}, {0, 2}, {1, 2}, {2, 0}, {2, 2}, {2, 1}
+ },
+ 5),
+ equalTo(1));
+ }
+}