|
| 1 | +# image_picker_for_web |
| 2 | + |
| 3 | +A web implementation of [`image_picker`][1]. |
| 4 | + |
| 5 | +## Browser Support |
| 6 | + |
| 7 | +Since Web Browsers don't offer direct access to their users' file system, |
| 8 | +this plugin provides a `PickedFile` abstraction to make access access uniform |
| 9 | +across platforms. |
| 10 | + |
| 11 | +The web version of the plugin puts network-accessible URIs as the `path` |
| 12 | +in the returned `PickedFile`. |
| 13 | + |
| 14 | +### URL.createObjectURL() |
| 15 | + |
| 16 | +The `PickedFile` object in web is backed by [`URL.createObjectUrl` Web API](https://developer.mozilla.org/en-US/docs/Web/API/URL/createObjectURL), |
| 17 | +which is reasonably well supported across all browsers: |
| 18 | + |
| 19 | +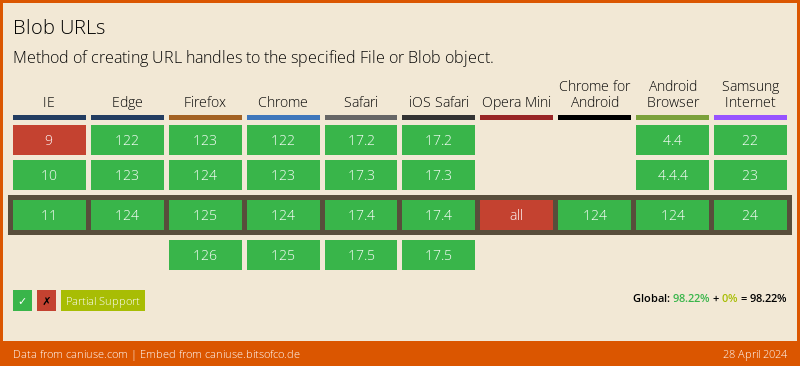 |
| 20 | + |
| 21 | +However, the returned `path` attribute of the `PickedFile` points to a `network` resource, and not a |
| 22 | +local path in your users' drive. See **Use the plugin** below for some examples on how to use this |
| 23 | +return value in a cross-platform way. |
| 24 | + |
| 25 | +### input file "accept" |
| 26 | + |
| 27 | +In order to filter only video/image content, some browsers offer an [`accept` attribute](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/accept) in their `input type="file"` form elements: |
| 28 | + |
| 29 | +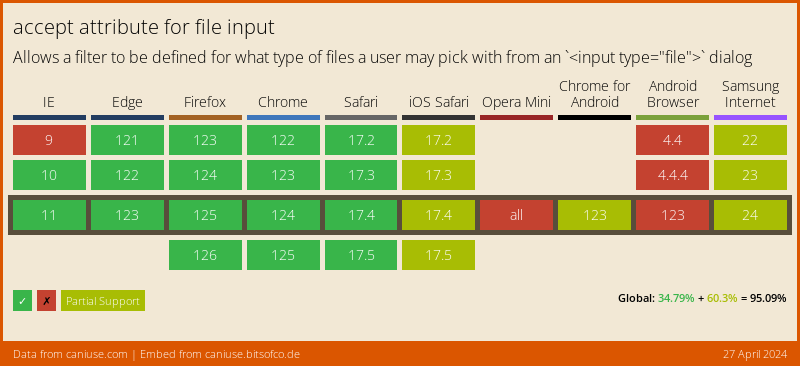 |
| 30 | + |
| 31 | +This feature is just a convenience for users, **not validation**. |
| 32 | + |
| 33 | +Users can override this setting on their browsers. You must validate in your app (or server) |
| 34 | +that the user has picked the file type that you can handle. |
| 35 | + |
| 36 | +### input file "capture" |
| 37 | + |
| 38 | +In order to "take a photo", some mobile browsers offer a [`capture` attribute](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/capture): |
| 39 | + |
| 40 | +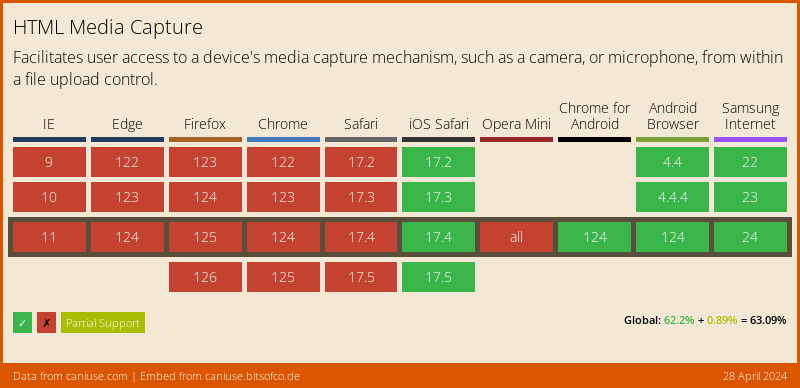 |
| 41 | + |
| 42 | +Each browser may implement `capture` any way they please, so it may (or may not) make a |
| 43 | +difference in your users' experience. |
| 44 | + |
| 45 | +## Usage |
| 46 | + |
| 47 | +### Import the package |
| 48 | + |
| 49 | +This package is an unendorsed web platform implementation of `image_picker`. |
| 50 | + |
| 51 | +In order to use this, you'll need to depend in `image_picker: ^0.6.7` (which was the first version of the plugin that allowed federation), and `image_picker_for_web: ^0.1.0`. |
| 52 | + |
| 53 | +```yaml |
| 54 | +... |
| 55 | +dependencies: |
| 56 | + ... |
| 57 | + image_picker: ^0.6.7 |
| 58 | + image_picker_for_web: ^0.1.0 |
| 59 | + ... |
| 60 | +... |
| 61 | +``` |
| 62 | + |
| 63 | +### Use the plugin |
| 64 | + |
| 65 | +You should be able to use `package:image_picker` _almost_ as normal. |
| 66 | + |
| 67 | +Once the user has picked a file, the returned `PickedFile` instance will contain a |
| 68 | +`network`-accessible URL (pointing to a location within the browser). |
| 69 | + |
| 70 | +The instace will also let you retrieve the bytes of the selected file across all platforms. |
| 71 | + |
| 72 | +If you want to use the path directly, your code would need look like this: |
| 73 | + |
| 74 | +```dart |
| 75 | +... |
| 76 | +if (kIsWeb) { |
| 77 | + Image.network(pickedFile.path); |
| 78 | +} else { |
| 79 | + Image.file(File(pickedFile.path)); |
| 80 | +} |
| 81 | +... |
| 82 | +``` |
| 83 | + |
| 84 | +Or, using bytes: |
| 85 | + |
| 86 | +```dart |
| 87 | +... |
| 88 | +Image.memory(await pickedFile.readAsBytes()) |
| 89 | +... |
| 90 | +``` |
| 91 | + |
| 92 | +[1]: https://pub.dev/packages/image_picker |
0 commit comments