Commit 62b1656
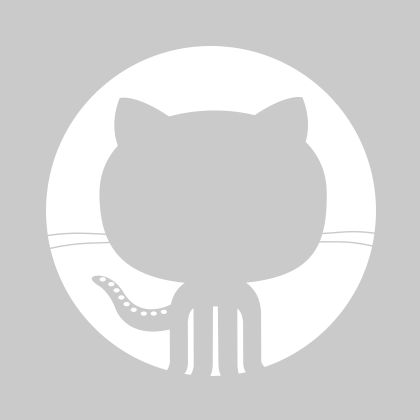
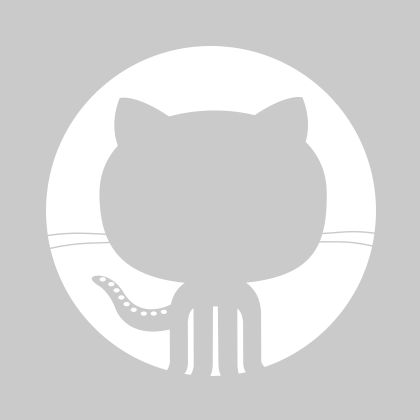
Connor Clark
Devtools-frontend LUCI CQ
1 parent 287be69 commit 62b1656
File tree
60 files changed
+4832
-3334
lines changed- front_end
- panels/lighthouse
- third_party/lighthouse
- locales
- report-assets
- test/webtests/http/tests/devtools/lighthouse
- resources
Some content is hidden
Large Commits have some content hidden by default. Use the searchbox below for content that may be hidden.
60 files changed
+4832
-3334
lines changedLines changed: 0 additions & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
71 | 71 |
| |
72 | 72 |
| |
73 | 73 |
| |
74 |
| - | |
75 | 74 |
| |
76 | 75 |
| |
77 | 76 |
| |
|
Lines changed: 821 additions & 824 deletions
Some generated files are not rendered by default. Learn more about customizing how changed files appear on GitHub.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 45 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 45 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 82 additions & 52 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 81 additions & 51 deletions
Large diffs are not rendered by default.
Lines changed: 16 additions & 4 deletions
Some generated files are not rendered by default. Learn more about customizing how changed files appear on GitHub.
Lines changed: 147 additions & 29 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2542 | 2542 |
| |
2543 | 2543 |
| |
2544 | 2544 |
| |
2545 |
| - | |
| 2545 | + | |
2546 | 2546 |
| |
2547 | 2547 |
| |
2548 | 2548 |
| |
| |||
2672 | 2672 |
| |
2673 | 2673 |
| |
2674 | 2674 |
| |
2675 |
| - | |
2676 |
| - | |
| 2675 | + | |
2677 | 2676 |
| |
2678 | 2677 |
| |
2679 | 2678 |
| |
| |||
2700 | 2699 |
| |
2701 | 2700 |
| |
2702 | 2701 |
| |
2703 |
| - | |
| 2702 | + | |
2704 | 2703 |
| |
2705 | 2704 |
| |
| 2705 | + | |
| 2706 | + | |
| 2707 | + | |
2706 | 2708 |
| |
2707 |
| - | |
2708 |
| - | |
| 2709 | + | |
| 2710 | + | |
| 2711 | + | |
| 2712 | + | |
| 2713 | + | |
2709 | 2714 |
| |
2710 | 2715 |
| |
2711 | 2716 |
| |
| |||
2714 | 2719 |
| |
2715 | 2720 |
| |
2716 | 2721 |
| |
2717 |
| - | |
2718 |
| - | |
| 2722 | + | |
2719 | 2723 |
| |
2720 |
| - | |
| 2724 | + | |
2721 | 2725 |
| |
2722 | 2726 |
| |
2723 | 2727 |
| |
| |||
3049 | 3053 |
| |
3050 | 3054 |
| |
3051 | 3055 |
| |
3052 |
| - | |
3053 |
| - | |
| 3056 | + | |
3054 | 3057 |
| |
3055 | 3058 |
| |
3056 | 3059 |
| |
3057 |
| - | |
3058 |
| - | |
| 3060 | + | |
3059 | 3061 |
| |
3060 | 3062 |
| |
3061 | 3063 |
| |
3062 |
| - | |
| 3064 | + | |
| 3065 | + | |
| 3066 | + | |
| 3067 | + | |
| 3068 | + | |
| 3069 | + | |
| 3070 | + | |
| 3071 | + | |
| 3072 | + | |
| 3073 | + | |
| 3074 | + | |
3063 | 3075 |
| |
3064 | 3076 |
| |
3065 | 3077 |
| |
3066 | 3078 |
| |
3067 | 3079 |
| |
3068 |
| - | |
| 3080 | + | |
3069 | 3081 |
| |
3070 | 3082 |
| |
3071 | 3083 |
| |
| |||
3074 | 3086 |
| |
3075 | 3087 |
| |
3076 | 3088 |
| |
3077 |
| - | |
3078 | 3089 |
| |
3079 | 3090 |
| |
3080 |
| - | |
| 3091 | + | |
| 3092 | + | |
| 3093 | + | |
| 3094 | + | |
| 3095 | + | |
| 3096 | + | |
| 3097 | + | |
| 3098 | + | |
| 3099 | + | |
| 3100 | + | |
3081 | 3101 |
| |
3082 | 3102 |
| |
3083 |
| - | |
| 3103 | + | |
| 3104 | + | |
| 3105 | + | |
3084 | 3106 |
| |
3085 | 3107 |
| |
3086 | 3108 |
| |
| |||
3106 | 3128 |
| |
3107 | 3129 |
| |
3108 | 3130 |
| |
3109 |
| - | |
3110 | 3131 |
| |
3111 | 3132 |
| |
3112 | 3133 |
| |
| 3134 | + | |
| 3135 | + | |
| 3136 | + | |
| 3137 | + | |
| 3138 | + | |
| 3139 | + | |
| 3140 | + | |
| 3141 | + | |
| 3142 | + | |
| 3143 | + | |
| 3144 | + | |
| 3145 | + | |
| 3146 | + | |
| 3147 | + | |
| 3148 | + | |
| 3149 | + | |
| 3150 | + | |
3113 | 3151 |
| |
3114 | 3152 |
| |
3115 | 3153 |
| |
| |||
4071 | 4109 |
| |
4072 | 4110 |
| |
4073 | 4111 |
| |
4074 |
| - | |
| 4112 | + | |
| 4113 | + | |
4075 | 4114 |
| |
4076 | 4115 |
| |
4077 |
| - | |
4078 |
| - | |
| 4116 | + | |
| 4117 | + | |
4079 | 4118 |
| |
4080 | 4119 |
| |
4081 | 4120 |
| |
| |||
4084 | 4123 |
| |
4085 | 4124 |
| |
4086 | 4125 |
| |
4087 |
| - | |
| 4126 | + | |
4088 | 4127 |
| |
4089 | 4128 |
| |
4090 | 4129 |
| |
| |||
4270 | 4309 |
| |
4271 | 4310 |
| |
4272 | 4311 |
| |
| 4312 | + | |
| 4313 | + | |
| 4314 | + | |
| 4315 | + | |
| 4316 | + | |
| 4317 | + | |
4273 | 4318 |
| |
4274 | 4319 |
| |
4275 | 4320 |
| |
4276 | 4321 |
| |
4277 | 4322 |
| |
4278 |
| - | |
4279 |
| - | |
4280 |
| - | |
4281 |
| - | |
4282 |
| - | |
4283 |
| - | |
4284 | 4323 |
| |
4285 | 4324 |
| |
4286 | 4325 |
| |
| |||
5010 | 5049 |
| |
5011 | 5050 |
| |
5012 | 5051 |
| |
| 5052 | + | |
| 5053 | + | |
| 5054 | + | |
| 5055 | + | |
| 5056 | + | |
| 5057 | + | |
| 5058 | + | |
| 5059 | + | |
| 5060 | + | |
| 5061 | + | |
| 5062 | + | |
| 5063 | + | |
| 5064 | + | |
| 5065 | + | |
| 5066 | + | |
| 5067 | + | |
| 5068 | + | |
| 5069 | + | |
| 5070 | + | |
| 5071 | + | |
| 5072 | + | |
| 5073 | + | |
| 5074 | + | |
| 5075 | + | |
| 5076 | + | |
| 5077 | + | |
| 5078 | + | |
| 5079 | + | |
| 5080 | + | |
| 5081 | + | |
| 5082 | + | |
| 5083 | + | |
| 5084 | + | |
| 5085 | + | |
| 5086 | + | |
| 5087 | + | |
| 5088 | + | |
| 5089 | + | |
| 5090 | + | |
| 5091 | + | |
| 5092 | + | |
| 5093 | + | |
| 5094 | + | |
| 5095 | + | |
| 5096 | + | |
| 5097 | + | |
| 5098 | + | |
| 5099 | + | |
| 5100 | + | |
| 5101 | + | |
| 5102 | + | |
| 5103 | + | |
| 5104 | + | |
| 5105 | + | |
| 5106 | + | |
| 5107 | + | |
| 5108 | + | |
| 5109 | + | |
| 5110 | + | |
| 5111 | + | |
| 5112 | + | |
| 5113 | + | |
| 5114 | + | |
| 5115 | + | |
| 5116 | + | |
| 5117 | + | |
| 5118 | + | |
| 5119 | + | |
| 5120 | + | |
| 5121 | + | |
| 5122 | + | |
| 5123 | + | |
| 5124 | + | |
| 5125 | + | |
| 5126 | + | |
| 5127 | + | |
| 5128 | + | |
| 5129 | + | |
| 5130 | + |
Lines changed: 4 additions & 4 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
323 | 323 |
| |
324 | 324 |
| |
325 | 325 |
| |
326 |
| - | |
327 |
| - | |
| 326 | + | |
| 327 | + | |
328 | 328 |
| |
329 | 329 |
| |
330 | 330 |
| |
| |||
393 | 393 |
| |
394 | 394 |
| |
395 | 395 |
| |
396 |
| - | |
397 |
| - | |
| 396 | + | |
| 397 | + | |
398 | 398 |
| |
399 | 399 |
| |
400 | 400 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
11 | 11 |
| |
12 | 12 |
| |
13 | 13 |
| |
14 |
| - | |
| 14 | + | |
15 | 15 |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
14 | 14 |
| |
15 | 15 |
| |
16 | 16 |
| |
17 |
| - | |
| 17 | + | |
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
|
Lines changed: 3 additions & 3 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 |
| - | |
| 7 | + | |
8 | 8 |
| |
9 | 9 |
| |
10 | 10 |
| |
11 |
| - | |
| 11 | + | |
12 | 12 |
|
0 commit comments