-
Notifications
You must be signed in to change notification settings - Fork 0
Weird values used for images
Stéphane Piette edited this page Mar 16, 2023
·
8 revisions
make a flask endpoint that returns an array of 6 points, each point having a random name, a random value, a short description and a long description. It should be compatible with char.js format
from flask import Flask, jsonify
import random
app = Flask(__name__)
@app.route('/points')
def get_points():
points = []
names = ["Point A", "Point B", "Point C", "Point D", "Point E", "Point F"]
short_descs = ["This is point A", "This is point B", "This is point C", "This is point D", "This is point E", "This is point F"]
long_descs = ["This is a longer description for point A", "This is a longer description for point B", "This is a longer description for point C", "This is a longer description for point D", "This is a longer description for point E", "This is a longer description for point F"]
for i in range(6):
point = {}
point['label'] = names[i]
point['value'] = random.randint(1, 10)
point['short_desc'] = short_descs[i]
point['long_desc'] = long_descs[i]
points.append(point)
return jsonify({'data': points})
if __name__ == '__main__':
app.run(debug=True)
write the javascript code to fetch those points and display them in a bar chart
// fetch the data from the Flask endpoint
fetch('/points')
.then(response => response.json())
.then(data => {
// extract the labels and values from the data
const labels = data.data.map(point => point.label);
const values = data.data.map(point => point.value);
// create the chart
const ctx = document.getElementById('myChart').getContext('2d');
const chart = new Chart(ctx, {
type: 'bar',
data: {
labels: labels,
datasets: [{
label: 'Values',
data: values,
backgroundColor: 'rgba(54, 162, 235, 0.5)',
borderColor: 'rgba(54, 162, 235, 1)',
borderWidth: 1
}]
},
options: {
scales: {
yAxes: [{
ticks: {
beginAtZero: true
}
}]
},
tooltips: {
callbacks: {
label: function(tooltipItem, data) {
const point = data.datasets[tooltipItem.datasetIndex].data[tooltipItem.index];
return data.datasets[tooltipItem.datasetIndex].label + ': ' + point + '\nShort description: ' + data.data[tooltipItem.index].short_desc + '\nLong description: ' + data.data[tooltipItem.index].long_desc;
}
}
}
}
});
})
.catch(error => {
console.error('Error fetching points:', error);
});
from those points, create an html list of card in bootstrap. each card should have as image the corresponding value of the point
// fetch the data from the Flask endpoint
fetch('/points')
.then(response => response.json())
.then(data => {
// create a list of cards
const cardsContainer = document.getElementById('cards-container');
data.data.forEach(point => {
const card = document.createElement('div');
card.classList.add('card', 'm-2');
const cardImg = document.createElement('img');
cardImg.src = `https://via.placeholder.com/150x${point.value}/000000/FFFFFF/?text=${point.label}`;
cardImg.alt = point.label;
cardImg.classList.add('card-img-top');
const cardBody = document.createElement('div');
cardBody.classList.add('card-body');
const cardTitle = document.createElement('h5');
cardTitle.classList.add('card-title');
cardTitle.textContent = point.label;
const cardText = document.createElement('p');
cardText.classList.add('card-text');
cardText.textContent = point.short_desc;
cardBody.appendChild(cardTitle);
cardBody.appendChild(cardText);
card.appendChild(cardImg);
card.appendChild(cardBody);
cardsContainer.appendChild(card);
});
})
.catch(error => {
console.error('Error fetching points:', error);
});
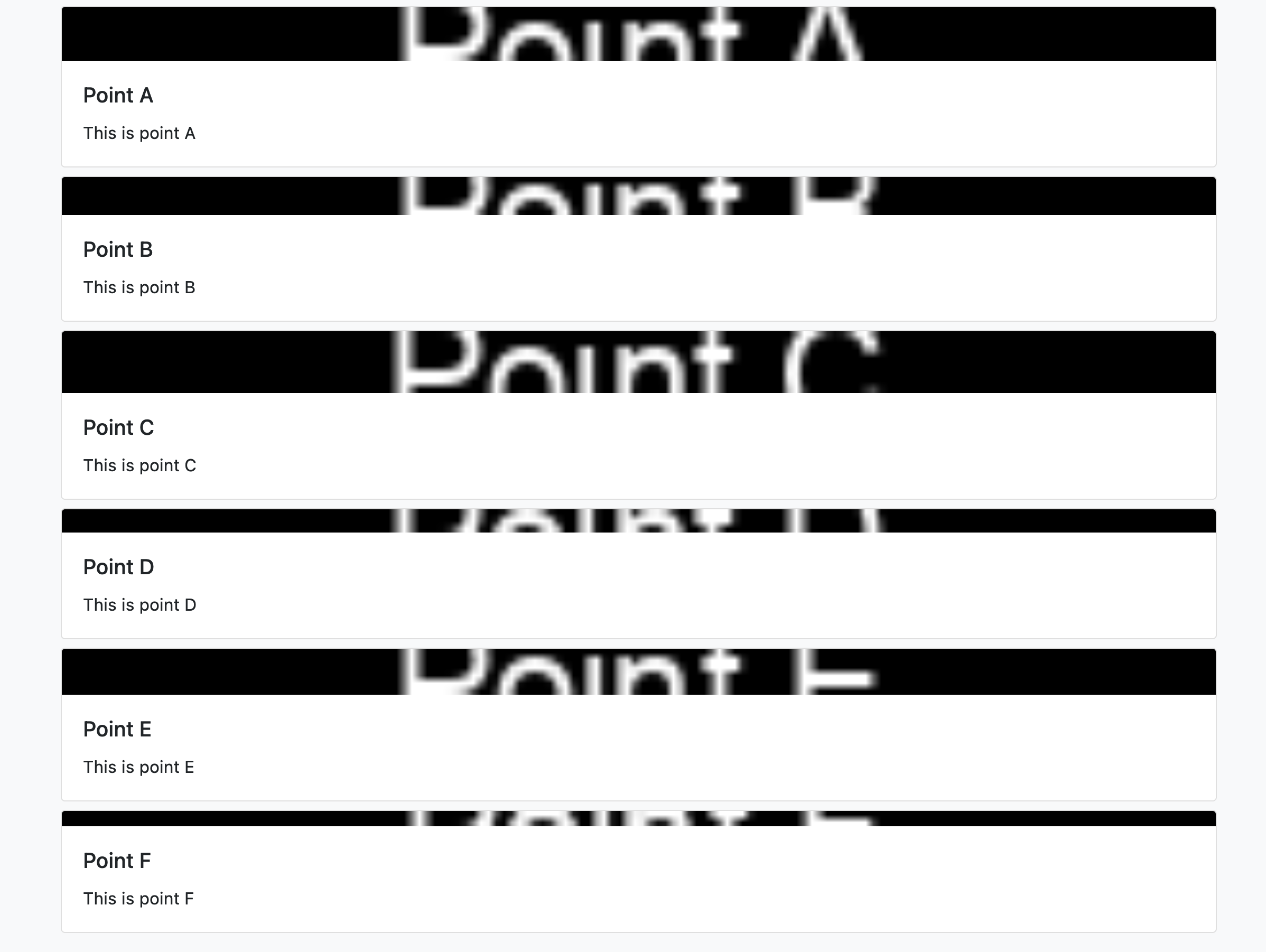
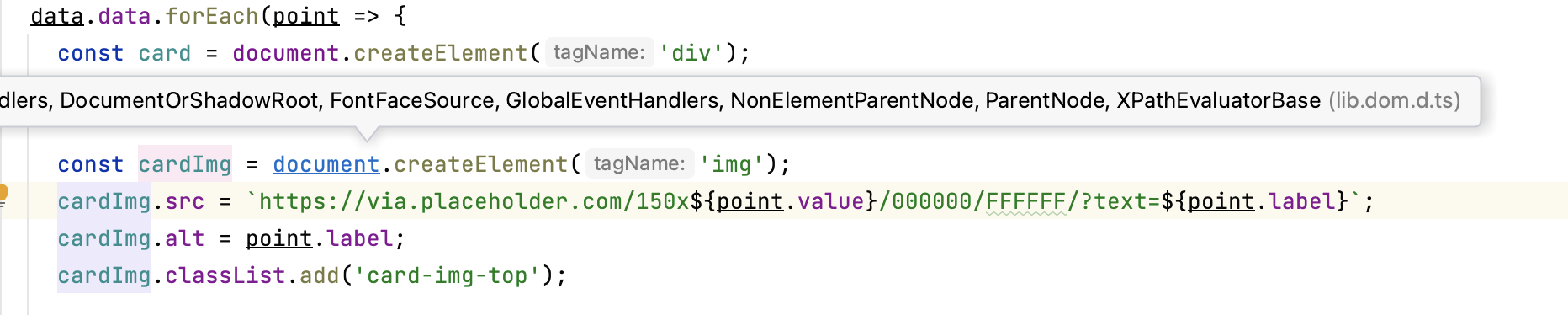
make the same as above but the card should be placed in a grid with 4 columns. The card images should have a dimension of 200*200
make the cards above clickable, being a link the details of the card